This might seem oddly specific, however its something I struggled with so I’m writing it down ;).
The Azure bit
Create the pipeline variable
- In an Azure pipeline, click on ‘Edit’ in the top right of the page:

- On the next page, click ‘Variable’
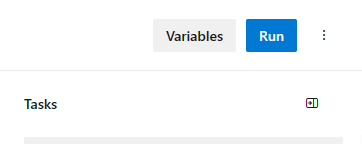
- Click ‘New variable’

- Create a new variable, the name must be appended with ‘CYPRESS_’, in this case we’re setting an ENVIRONMENT variable:

I’ve also checked the option which will allow users to override the value when running the pipeline. I’d recommend doing this in case you want to run tests on a lower environment than production.
The Cypress bit
We want to be able to change which base URL we use depending on the environment variable set in our azure pipeline.
Within our Cypress project/Cypress within our project, create a new file in cypress/support called setBaseUrl.js.

In this file, add the following code:
Cypress.Commands.add('setBaseUrl', () => {
const environment = Cypress.env('ENVIRONMENT')
if(environment == 'test'){
Cypress.config('baseUrl', 'https://www.google.com')
} else if(environment == 'staging') {
Cypress.config('baseUrl', 'https://www.bing.com')
} else {
Cypress.config('baseUrl', 'https://www.yahoo.com')
}
});
The code above fetches the CYPRESS_ENVIRONMENT variable we set on the azure pipeline, and sets the baseUrl for the correct environment.
Next we need to import the new command, open e2e.js, and add the following:
import './setBaseUrl'

This will make the command available to us in our tests.
Next we’ll create a test which will use the command which, in turn sets the baseUrl. Create a new file in cypress/e2e called test.cy.js:
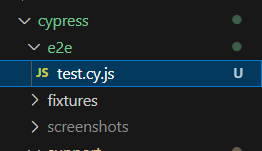
Add the following code, which calls the setBaseUrl command we have created and visits the page root:
describe('Visiting the correct URL', () => {
beforeEach(() => {
cy.setBaseUrl()
cy.visit('/')
})
it('is reachable', () => {
// Confirm title
cy.get('body')
})
})
Currently our environment variable in Azure is set to ‘production’, so we should find ourselves on ‘https://www.yahoo.com’ when we run the tests.
Fire up Cypress and run the test.cy.js test we have created:

PERFECT!!!
Just to make sure, lets change the environment variable to ‘test’ and re-run, we should see Google:

And lastly, change the environment variable on the Azure pipeline to ‘staging’, we should see Bing:

YES! All done!