Learn how to create a basic monorepo containing multiple next.js apps.
Creating the project
In terminal, create a new directory for our monorepo, and set up a basic package.json using yarn:
mkdir next-mono-post
cd next-mono-post/
yarn init -y
Creating workspaces
Open the package.json file in your IDE, delete everything then add the below:
{
"name": "next-mono-post",
"private": true,
"version": "1.0.0",
"workspaces": [
"apps/*",
"packages/config/*",
"packages/shared/*"
],
"engines": {
"node": ">=20.0.0"
},
"packageManager": "yarn@1.22.21"
}
The workspaces array tells the package manager where our workspaces are in our monorepo:
- apps/* – will contain all of our independent next.js apps.
- packages/config/* – will contain reusable packages (formatting, linting…)
- packages/shared/* – will contain shared code to be used across our next.js apps (UI components)
Adding Next.js apps
Create a new apps directory at the root of our monorepo:
mkdir apps
Cd into the apps directory and use yarn to generate a few next apps, when generating the apps, you’ll be ok in this example to use the default config:
cd apps
yarn create next-app app1
yarn create next-app app2
yarn create next-app api
Your project structure should now look like this:
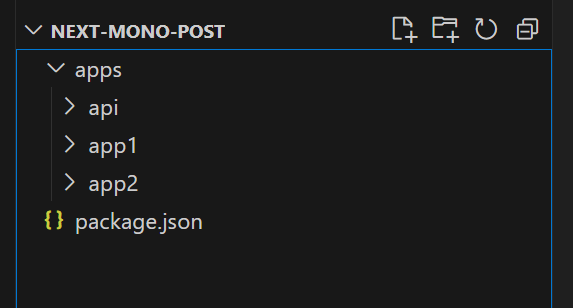
Updating the pages
So that we can confirm we are looking at the right apps, lets update the homepages of each:
- Open apps/app1/app/page.tsx
- Delete everything from the file
- Replace with the following:
export default function Home() {
return (
<div>
<h1>App1</h1>
</div>
);
}
Do the same in apps/app2/app/page.tsx and apps/api/page.tsx, replacing the string in the <h1> tag to App2 and Api respectively.
Running the Apps
To run each of the apps, cd into the app directory (apps/api, apps/app1, apps/app2) and run yarn dev, you wont need to manually specify a port, if a port is already in use yarn will assign a different one.